1.对象内存布局
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27
| class A1: public Base { class Base { class Base_vtable* class Base_member { int m_iVal1; char m_cVal2; }; }; class A1_member { float m_fVal1; char* m_pcVal2; }; };
class Base_vtable { int64 fun1; int64 fun2; };
|
1.1 基本类
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30
| #include <iostream>
class A1 { public: A1(int x, char y, short z) { this->x = x; this->y = y; this->z = z; } void show() { std::cout << x << std::endl; std::cout << y << std::endl; } private: int x; char y; short z; };
int main() {
A1 a1(3, 5, 7); a1.show();
return 0; }
|
对于基本类,从对象创建的地方找到构造函数,根据结构偏移使用情况来确定边界和成员类型,并在之后分析过程中不断修改完善。
结构体定义模板
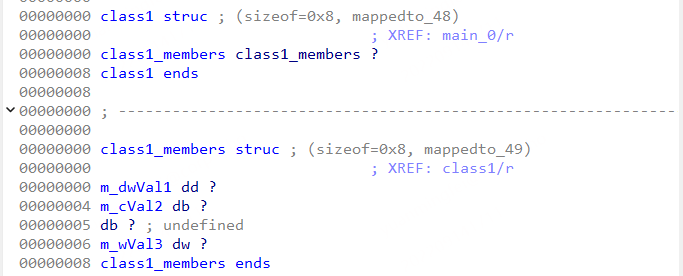
1.2 组合类
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61
| #include <iostream>
class A2 { public: A2(float x, float y) { this->x = x; this->y = y; } float GetX() { return x; } float GetY() { return y; } private: float x; float y; };
class A1 { public: A1(int x, char y, short z) :a2(3.2, 4.3) { this->x = x; for (int i = 0; i < 20; i++) { this->y[i] = i+1; } this->z = z; } void show() { std::cout << x << std::endl; std::cout << y << std::endl;
std::cout << y[5] << std::endl; std::cout << a2.GetX() << std::endl; std::cout << a2.GetY() << std::endl; } private: int x; char y[20]; short z; A2 a2; };
int main() {
A1 a1(3, 5, 7); a1.show();
return 0; }
|
组合类在分析的时候可以看见很明显的特征,就是在class1的构造函数中会将 this + x 的地址传入class2的构造函数中进行初始化,这个偏移x的位置就是class2的起始位置。

结构体定义模板
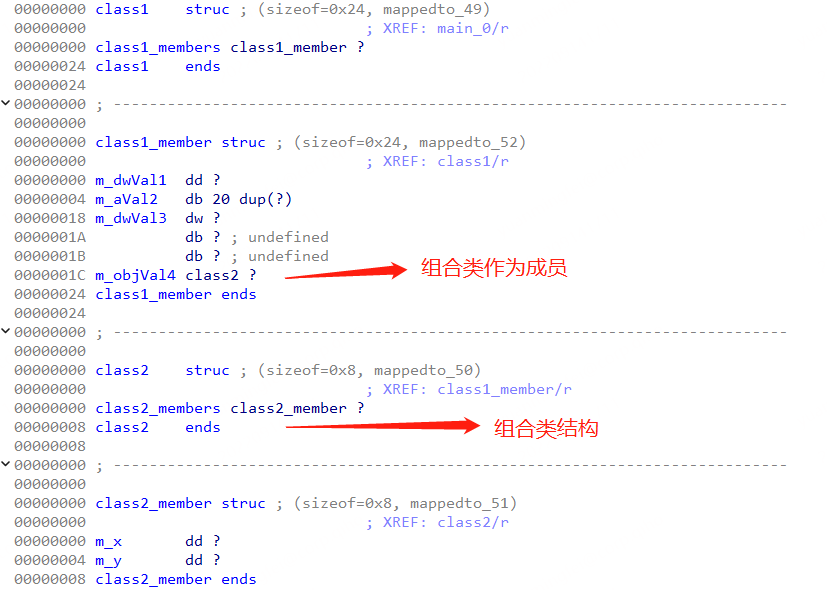
1.3 单继承类
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52
| #include <iostream>
class A2 { public: A2(int a, int len) { this->a = a; b = new char(len); strcpy(b, "Base"); } ~A2() { if (this->b) { free(this->b); this->b = NULL; } } protected: int a; char *b; int arr[5]; };
class A1 : public A2 { public: A1(int a, short c) :A2(8, 12) { this->a = a; this->c = c; } int encrypt(int key) { return key + b[3] + b[5] * arr[1] / a + c; } private: int a; short c; };
int main() {
A1 a(3, 5); std::cout << a.encrypt(10) << std::endl;
return 0; }
|
对于继承关系的类识别,可以看构造函数中是否有将原始this指针直接传递给父类构造函数的操作。实际上在不含有虚函数的继承关系中,可以把子类和父类合并来看待。普通继承关系实际上就是把父类的成员原封不动的拷贝过来,作为子类对象结构开头的一部分。
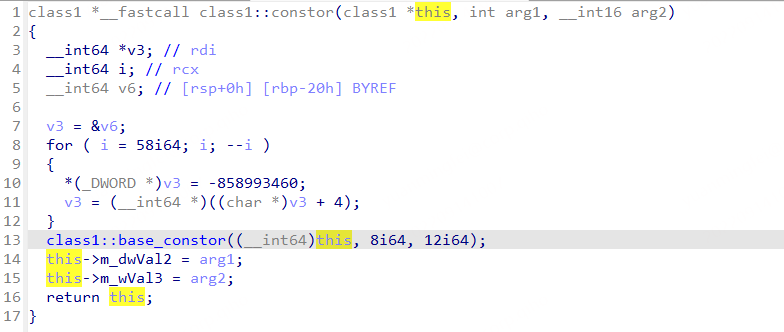
结构体定义标准模板
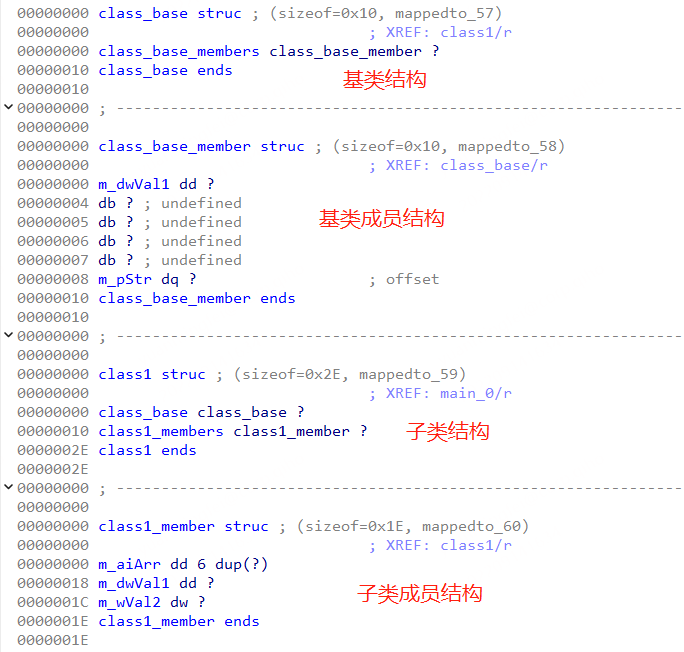
1.4 多继承类
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67
| #include <iostream>
class TopBase { public: TopBase(int id, char* name) { this->dwId = id; this->sName = name; } protected: int dwId; char* sName; };
class Mid1 :public TopBase { public: Mid1(int age) :TopBase(1, "jack") { this->age = age; } protected: int age; };
class Mid2 :public TopBase { public: Mid2(char high) :TopBase(2, "tom") { this->high = high; } protected: char high; };
class Bottom :public Mid1, Mid2 { public: Bottom(char* grade, char* name) :Mid1(20), Mid2(185) { this->grade = grade; this->sName = name; } void show() { std::cout << age << std::endl; } private: char* grade; char* sName; };
int main() { Bottom obj("100", "xiaoming"); obj.show();
return 0; }
|
继承关系呈现菱形结构,就是有两个类继承自同一个类,同时又有另外一个类同时继承自这两个。多重继承会在子类中出现多个父类的members结构,并根据先后顺序排列,可以从最上层依次分析,逐渐向下递进,直至分析出继承树结构。
多重继承结构体定义标准模板
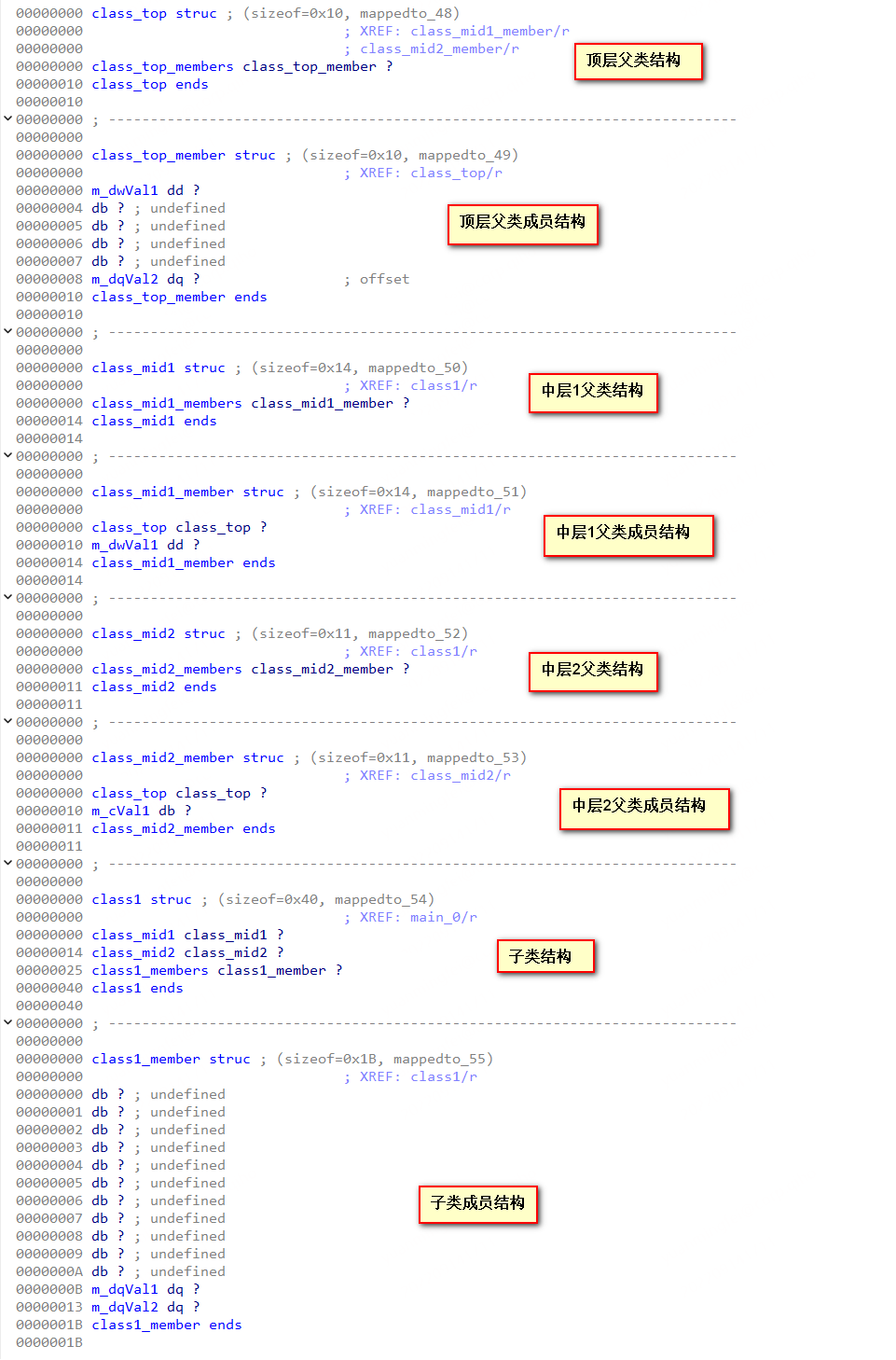
1.4 虚表类
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80
| #include <iostream>
class Base1 { public: Base1(char* str) { int len = strlen(str); pa = new char[len]; strcpy(pa, str); } virtual void show() { std::cout << "Base1" << std::endl; std::cout << pa << std::endl; } virtual ~Base1() { if (pa) { free(pa); pa = NULL; } } protected: char* pa; };
class Base2 { public: Base2(int a) { this->a = a; } virtual void show() { std::cout << a << std::endl; } virtual ~Base2() { std::cout << "destory Base2" << std::endl; } protected: int a; };
class A1 :public Base1, public Base2 { public: A1() :Base1("Hello"), Base2(5) { strcpy(buf, "World"); this->a = a; } virtual void show() { std::cout << "A1" << std::endl; std::cout << buf << std::endl; } virtual ~A1() { std::cout << "A1 destory" << std::endl; } private: char buf[10]; int a; };
int main() { A1 a1; Base1* p = &a1; p->show();
return 0; }
|
虚表指针属于基类,不属于子类,子类只是将其继承过来。所以在子类的结构中应包含父类的结构,在父类的结构中包含虚表结构和父类成员结构。多个父类结构在子类结构中顺序排列。
多重继承虚表结构定义标准模板
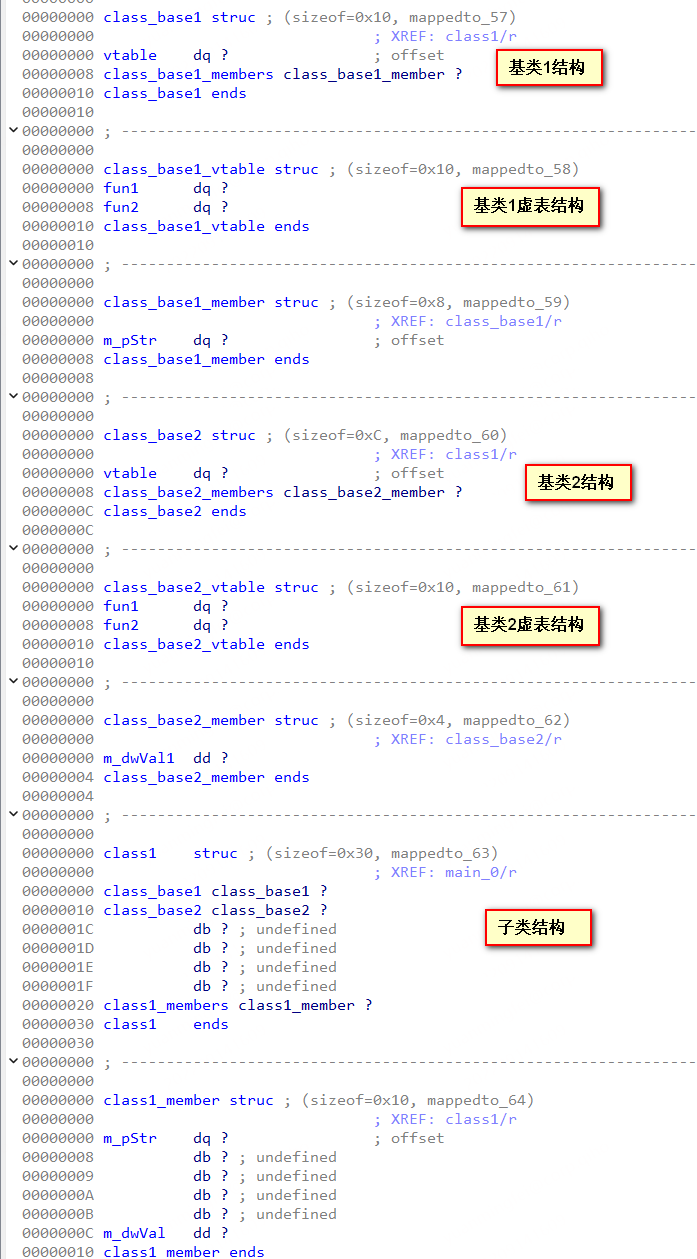
2.对象创建方式
2.1 局部对象
局部对象是最常见的创建方式,反汇编可以看见直接将栈内存地址传给构造函数。

2.2 堆对象
对象存在new关键字动态申请出来的内存中,反汇编可以看见直接将new得到的地址传入构造函数。

2.3 全局对象和静态对象
对于全局对象和静态对象,都是在.data段分配内存,区别就是静态对象说明对象作用域在当前文件中。识别全局对象的调用可以寻找 lea rcx, qword_xxx 指令。
全局对象的构造函数在main函数前完成,在 crt->initterm 中完成初始化。
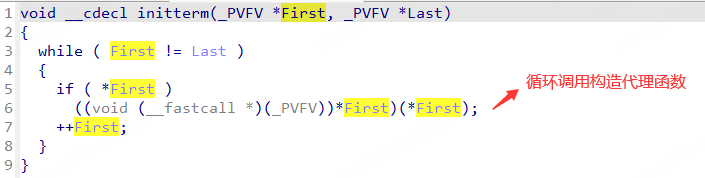
可以在First到Last区间内找到所有的代理构造函数地址,代理构造函数负责调用构造函数完成初始化以及调用 atexit 函数来注册析构函数,以便在main函数结束后调用。
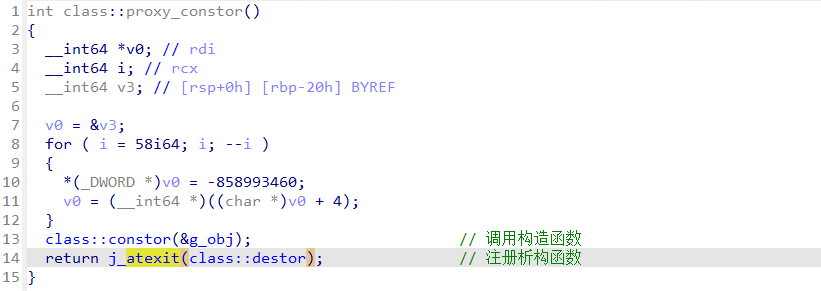
2.4 参数对象
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40
| #include <iostream>
class A1 { public: A1(int a, char b) { this->m_dwVal1 = a; this->m_cVal2[5] = b; } A1(const A1& c) { this->m_dwVal1 = c.m_dwVal1; strcpy(this->m_cVal2, c.m_cVal2); } void show() { std::cout << this->m_dwVal1 << std::endl; std::cout << this->m_cVal2[8] << std::endl; } public: int m_dwVal1; char m_cVal2[20]; };
void Show(A1 arg) { std::cout << arg.m_cVal2[3] << std::endl; arg.show(); }
int main() {
A1 a1(3, 5); Show(a1);
return 0; }
|
调用拷贝构造函数或直接复制对象空间内存来在参数区构造一个新的对象,并将新对象的内存首地址给调用的函数。
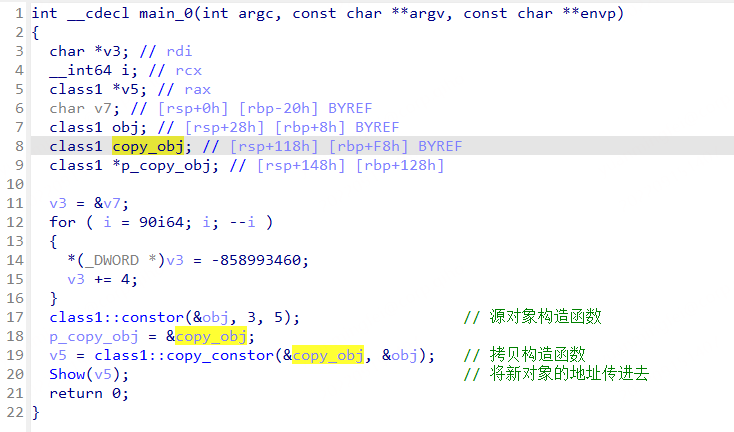
2.5 返回对象
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38
| #include <iostream>
class A1 { public: A1(int a, char b) { this->m_dwVal1 = a; this->m_cVal2[5] = b; } A1(const A1& c) { this->m_dwVal1 = c.m_dwVal1; strcpy(this->m_cVal2, c.m_cVal2); } void show() { std::cout << this->m_dwVal1 << std::endl; std::cout << this->m_cVal2[8] << std::endl; } public: int m_dwVal1; char m_cVal2[20]; };
A1 Show() { A1 a1(3, 5); return a1; }
int main() {
A1 a1 = Show(); return 0; }
|
对象作为返回值传递会先在调用之前将缓冲区首地址提前传进去(即使那个函数没有参数)。
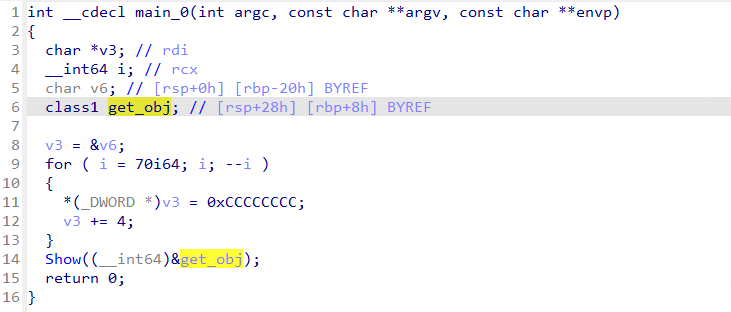
在函数里面调用构造函数,在函数返回前根据传进来的上一个函数缓冲区的首地址和当前这个对象的首地址作为参数来调用拷贝构造函数。
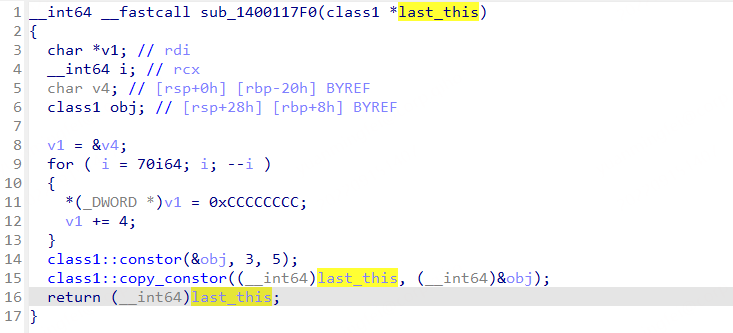